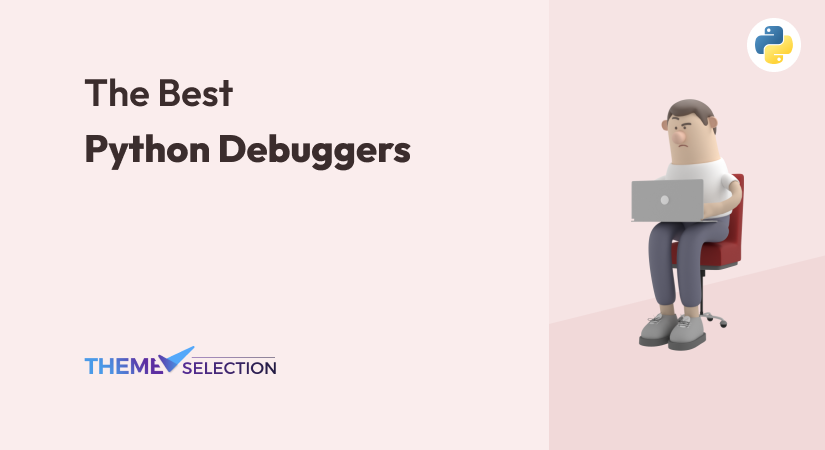
Facing issues while programming with Python? Well, worry not, and check out the best Python Debuggers to simplify your development with Python.
Table Of Content
What is A Debugger?
Essentially, a debugger for Python is a tool that gives you a way to, let’s say, open up the application in a certain spot so you can have a look at your variables, call stack, or whatever you need to see, set conditional breakpoints, step through the source code line by line, etc.
It’s like opening the hood of your car to see where the smoke is coming from, searching part by part to identify the problem. If you’re working with Python, not only can you look through the code during debugging, but you can also run the code that’s written in the command line or even affect the process by changing the variables’ value.
In this article, we are gonna talk about the best Python Debuggers.
Python debugging refers to identifying and fixing errors in Python code. This can include finding syntax, semantic, and runtime errors that prevent the code from functioning. Various techniques and tools are available for debugging Python code.
Why Do You Need It?
A debugger allows developers to pause the execution of their code and inspect variables, expressions, and the program’s state at any given point. This makes it easier to isolate the source of an error and understand how the code is behaving.
Without a debugger, finding and fixing bugs can be time-consuming and challenging, especially as the code grows in size and complexity. Following are some of the advantages of using Python Debuggers:
- Saves time
- Improves code quality
- Comprehensive understanding of the code
- Debug complex errors with ease
- Improves team collaboration
- Improves efficiency & development
Python Debugging Steps:
You can simplify the coding with the help of the best Python Debuggers by following some steps. The typical process involved in debugging Python code includes the following steps:
- Identify the error: This can be done by reading error messages, reviewing log files, or testing the code and observing any unexpected behavior.
- Reproduce the error: To debug the code, you need to be able to reproduce the error consistently.
- Isolate the source of the error: Once you have reproduced the error, you need to isolate the source of the problem by using techniques such as adding print statements, using a debugger, or examining stack traces.
- Form a hypothesis: From the information gathered, form a hypothesis about what might be causing the error.
- Test the hypothesis: Use your hypothesis to guide your debugging process by making changes to the code and observing the results.
- Repeat the process: If the hypothesis is incorrect, repeat the process from Step 3 until the error is fixed.
- Verify the fix: Once you have fixed the error, verify that the code is working correctly by repeating the steps that initially caused the error.
- Document the fix: Document the error, the steps you took to fix it, and any relevant information that will help you or others avoid similar issues in the future.
Python is not difficult to debug. It has a simple syntax and easy-to-understand error messages. Besides, it provides built-in debugging tools like PDB and offers integration with popular IDEs and third-party Python debuggers, which can greatly simplify the debugging process.
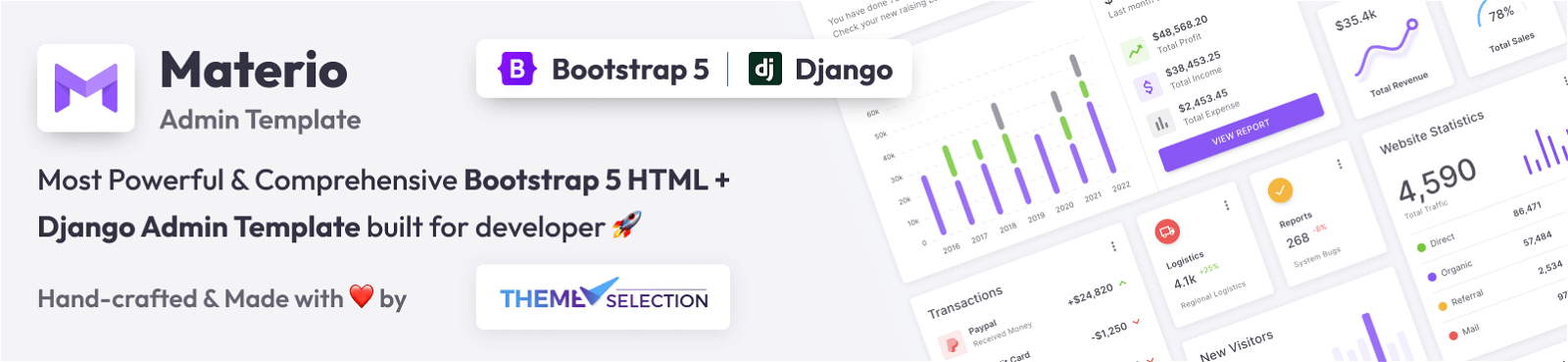
The Best Python Debuggers:
Debugging tools are at the heart of any programming language. And as a developer, it’s hard to make progress and write clean code unless you know your way around these tools.
With the growing popularity of Python and its increasing use in various industries, there has been a continuous effort to improve and enhance the Python debugging tools and techniques available to Python developers.
Today, a rich ecosystem of Python debuggers tools and techniques is available to help Python developers. Well, this collection will help you get familiar with the best Python debugging tools.
Let’s check them out.
Python exceptions are a mechanism for handling errors and exceptional conditions that may occur during the execution of a Python program. When an error or an exceptional condition occurs, Python raises an exception, which interrupts the normal flow of the program and transfers control to a special block of code called an exception handler.
There are two types of exceptions:
– Built-in Exceptions.
– User-Defined Exceptions.
To handle exceptions, you can use a <strong>try-except</strong>
block. The code that might raise an exception is placed inside the try
block, and the corresponding exception handler code is placed inside the except
block. If an exception occurs within the try
block, Python looks for a matching except
block that can handle the exception. In case a match is found, the code inside the except
block is executed, and the program continues to run normally. If no matching except
block is found, the exception propagates up the call stack until it is handled or the program terminates.
PDB – Built-in Debugger of Python
The first one in this list of Python Debuggers is nonother than Python’s built-in Debugger PDB. The module pdb
defines an interactive source code debugger for Python programs. It is a built-in part of the standard library that Python comes with during installation on your work tool. Besides, this Python debugging tool gives you several other commands you can use when testing your program.
Furthermore, it supports setting (conditional) breakpoints and single stepping at the source line level, an inspection of stack frames, source code listing, and evaluation of arbitrary Python code in the context of any stack frame.
Additionally, it supports post-mortem debugging and can be useful under program control. Besides, it also provides a command-line interface that allows developers to pause the execution of a program, inspect variables, and step through the code to identify and resolve issues. This makes it one of the considerable Python debuggers.
Prerequisites
To understand the process of debugging, you need to have:
- Python installed on your local machine
- Knowledge of Python
How To Use Python Debugger Pdb?
To use the Python debugger (pdb), follow these steps:
- Import pdb into your script or run it from the command line using the “
-m pdb
” flag followed by the script’s name you want to debug. - Activate the debugger by setting breakpoints at specific lines using the “
break
” command. This allows you to pause execution and inspect the program’s state at that point. - Once paused, navigate through the code and perform debugging operations using various commands. For example:
- Use the “
step
” command to move to the next line of code. - Utilize “
continue
” to resume execution until the next breakpoint is reached. - Employ “
print
” to display the value of a variable.
- Use the “
- Explore other pdb commands to assist in navigating, inspecting variables, and resolving bugs in your Python programs.
Important pdb Commands:
Following are some of the most important debugger commands that you will need while using pdb Python Debugger. Most commands can be abbreviated to one or two letters as indicated; e.g. h(elp)
means that either h or help can be used to enter the help command (but not he or hel, nor H or Help or HELP)
<strong>h</strong>
– Without argument, print the list of available commands.<strong>p</strong>
– Print the value of an expression.up
– In the case of nested function calls, it will move you up into the function that called your function.pp
– Pretty-print the value of an expression.n
– Continue execution until the next line in the current function is reached or it returns.<strong>s</strong>
– Execute the current line and stop at the first possible occasion<strong>c </strong>
– Continue execution and only stop while encountering the breakpoint<strong>unt </strong>
– Continue execution until the line with a number greater than the current one is reached. With a line number argument, continue execution until a line with a number greater or equal to that is reached.
Refer to the official site for the full list of Debugger Commands.
Python DevTool
The python-devtools project is a collection of Python development and Python debuggers tools created by Samuel Colvin. These tools are designed to simplify common development tasks and make it easier for developers to write high-quality Python code.
The project includes several different modules that can be used individually or together. Some of the most notable modules include:
- Debugging: The debugging module provides a number of tools for debugging Python code, including a debugging shell, a code profiler, and a tracer.
- Logging: The logging module provides an easy-to-use logging system that can be used to log messages at different levels of severity.
- Testing: The testing module provides a simple testing framework that makes it easy to write unit tests for Python code.
- Profiling: The profiling module provides tools for profiling the performance of Python code.
- Benchmarking: The benchmarking module provides tools for benchmarking the performance of different Python implementations.
Overall, the Python-devtools project is a useful collection of tools for anyone working with Python. Whether you’re a beginner or an experienced developer, this set of Python debuggers can help you write better code, debug more effectively, and optimize the performance of your Python applications.
Requires:
- Python >=3.7
License:
- MIT
How To Use Python Devtools?
Python-devtools provides a number of tools that are useful during Python development, including debug()
an alternative to <strong>print()</strong>
which formats the output in a way that should be easier to read than print
as well as giving information about which file/line the print statement is on and what value was printed.
To use the devtools
package from PyPI, follow these steps:
- Install
devtools
by running<strong>pip install devtools</strong>
in your terminal or command prompt. - Import the package into your Python script or interactive session using
<strong>import devtools</strong>
. - Use the
devtools
package for various development tasks:- For debugging, use the
debug
function to print and inspect variables during runtime. - Use the
debug
function to inspect objects and print their contents. - Trace function calls by decorating the desired function with
@trace
.
- For debugging, use the
- Explore the
devtools
documentation or source code for additional features and their usage.
Remember that the <strong>devtools</strong>
package for Python is distinct from the devtools
package used in JavaScript development. Refer to the specific Python package documentation to ensure proper usage.
Important Commands:
The devtools
package offers important commands for various development tasks:
<strong>debug()</strong>
: Print and inspect variables during runtime for debugging purposes.<strong>debug_pprint()</strong>
: Similar to<strong>debug()</strong>
, but provides formatted and readable output usingpprint
.<strong>trace()</strong>
: Decorator for tracing function calls, providing detailed logs of function entry, exit, and arguments.<strong>log()</strong>
: Log messages with different severity levels, such as informational, warning, or error.<strong>profile()</strong>
: Profile your code and measure execution time to identify performance bottlenecks.<strong>timecall()</strong>
: Measure the execution time of a function and display the elapsed time.
Refer to the devtools documentation or source code for more commands and their specific usage.
IPython
IPython is not primarily a Python debugger but rather an enhanced interactive shell for Python. However, IPython does provide some debugging capabilities that can be useful for Python programming. Here are a few ways IPython can assist you with debugging:
- Interactive Debugging: it allows you to interactively debug your code using its debugging magic commands. You can set breakpoints, step through your code line by line, inspect variables, and execute code snippets to troubleshoot issues.
- Post-mortem Debugging: If your code encounters an error or exception, IPython provides a post-mortem debugging feature that allows you to investigate the state of your program at the point of failure. This can help you identify the cause of the error and fix the problem.
- Code Profiling: IPython includes profiling tools that can help you analyze the performance of your code. You can measure the execution time of specific code sections, identify bottlenecks, and optimize your code for better performance.
- Tab Completion and Documentation: IPython offers powerful tab completion functionality, allowing you to explore the available methods, attributes, and functions of objects. It also provides access to inline documentation, enabling you to quickly retrieve information about Python objects and modules.
- Experimentation and Prototyping: IPython’s interactive shell provides a convenient environment for experimentation and prototyping. You can quickly test code snippets, explore data, and iterate on ideas without the need to write a complete script or program.
It’s important to note that while IPython can be helpful for debugging, it’s not a replacement for dedicated Python debuggers like pdb (Python Debugger) or integrated development environments (IDEs) that offer advanced debugging features.
However, IPython’s debugging capabilities can be valuable in scenarios where you’re already using IPython for interactive work and want to perform basic debugging tasks without switching to a dedicated debugger.
How To Use iPython?
To use IPython for debugging, you can follow these steps:
- Install IPython using pip by running
<strong>$ pip install ipython</strong>
command in your terminal - launch IPython by running the
<strong>ipython</strong>
command in your terminal or command prompt. This will open the IPython interactive shell. - set a breakpoint in your code, you can use the
<strong>%debug</strong>
magic command in IPython. For example, if you have a script named<strong>my_script.py</strong>
, you can run it with debugging enabled by entering the command:%debug run my_script.py
- Once the program hits a breakpoint, you can use various debugging commands to navigate through the code and inspect variables.
- If an exception occurs in your code and you want to debug it using IPython, you can use the
%pdb 1
or%pdb on
magic command to enable post-mortem debugging.- Now, it will automatically start on each unhandled exception
- You can turn this behavior off again with
%pdb 0
or<strong>%pdb off</strong>
. Running<strong>%pdb</strong>
without any argument will toggle the automatic debugger on and off.
IPython provides additional features and commands that can be explored in its documentation or by using the <strong>%help</strong>
command within IPython.
Commonly Uses Command:
n
or<strong>next</strong>
: Execute the next line of code.<strong>s</strong>
or<strong>step</strong>
: Step into the next function call.c
or<strong>continue</strong>
: Continue execution until the next breakpoint or the program finishes.l
or<strong>list</strong>
: Show the current section of the code.<strong>p <variable></strong>
: Print the value of a variable.q
or<strong>quit</strong>
: Quit the debugger.
You can type these commands directly in the IPython prompt to control the debugger.
Trepan3
Another one from this list of Python debuggers is python3-trepan
. It is a Python 3-based debugging tool that offers advanced capabilities for identifying and resolving bugs in Python programs.
It provides an interactive command-line interface, allowing developers to step through code, set breakpoints, and examine variables. With <strong>python3-trepan</strong>
, developers can gain deeper insights into program behavior and control the execution flow.
Following are some of the debugging features it offers:
- Interactive Debugging: The debugger provides an interactive shell for examining and manipulating the program state during runtime. It allows variable inspection, expression evaluation, and a deeper understanding of code behavior.
- Breakpoints: Pause program execution at specific locations to examine variable states and step through the code line by line. Set breakpoints anywhere in the code, including functions, classes, or modules.
- Stepping through Code: Gain full control over execution flow by stepping through code one line at a time. Easily trace function calls, skip statements, and exit functions to analyze code behavior.
- Variable Inspection: Inspect variable values at any program point, helping identify issues like incorrect assignments or unexpected values.
- Code Evaluation: Evaluate expressions and run Python code within the program’s context. Test hypotheses, explore code behavior, and gain insights into complex program states.
- Conditional Breakpoints: Set breakpoints to trigger based on specific conditions. Pause program execution when certain variables have specific values or follow particular code paths.
- Post-mortem Debugging: Support for post-mortem debugging
- Integration with Editors: Seamless integration with popular text editors and IDEs like Vim, Emacs, and PyCharm, allows for convenient code debugging within the preferred development environment.
Such features make it one of the best Python Debuggers.
How to use Trepan3k?
The debugger supports features such as conditional breakpoints, post-mortem debugging, and integration with popular editors, enhancing the debugging experience. By leveraging its interactive shell and code evaluation capabilities, developers can efficiently analyze and rectify issues, improving the overall quality and reliability of Python applications.
Commonly Used Commands:
break
orb
: Sets a breakpoint at a specific line of code.continue
orc
: Resumes program execution until the next breakpoint or the end.disable
: Disables specified breakpoints or all breakpoints.display
: Evaluates and displays an expression each time the program stops.down
: Moves the current frame down in the call stack.enable
: Enables specified breakpoints or all breakpoints.finish
: Continues execution until the current function returns.ignore
: Sets the number of times a breakpoint is ignored before stopping.info
: Provides information about breakpoints, stack frames, and variables.jump
: Jumps to a specified line number in the current file.restart
: Restarts the debugged program.return
orr
: Continues execution until the current function returns.run
: Starts or restarts the debugged program.step
ors
: Steps through the program, executing one line at a time.- For further commands, check the official Guide
Pdp++
This module is an extension of the pdb module of the standard library. It is meant to be fully compatible with its predecessor, yet it introduces a number of new features to make your debugging experience as nice as possible.
PDP++ is a powerful Python debugger built on top of the standard pdb module. It provides advanced features and enhancements to facilitate efficient debugging and analysis of Python code. With PDP++, developers can gain greater insight into the execution flow, variables, and stack traces, thereby aiding in the identification and resolution of bugs and issues in their software.
This Python Debugger offers the following features.:
- colorful TAB completion of Python expressions (through fancycompleter)
- optional syntax highlighting of code listings (through Pygments)
- sticky mode
- several new commands to be used from the interactive
(Pdb++)
prompt - smart command parsing (hint: have you ever typed
r
orc
at the prompt to print the value of some variable?) - additional convenience functions in the
pdb
module, to be used from your program
pdb++
is meant to be a drop-in replacement for pdb
. If you find some unexpected behavior, please report it as a bug.
How To Use PDP++?
Since <strong>pdb++</strong>
is not a valid package name the package is named <strong>pdbpp</strong>
:
<strong>$ pip install pdbpp</strong>
<strong>pdb++</strong>
is also available via conda:
$ conda install -c conda-forge pdbpp
Alternatively, you can just put pdb.py
somewhere inside your PYTHONPATH
.
Important Commands:
PDP++ provides several additional interactive commands beyond the standard pdb module. Here are some important commands you can use during debugging:
sticky
: Toggles persistent breakpoints across debugger runs.where
orw
: Displays the stack trace for the current execution context.args
: Shows arguments passed to the current function or frame.interact
: Temporarily suspends debugger, allowing interactive shell access.longlist
orll
: Displays an extended view of source code.jump <line>
: Jumps to a specific line number in the code.enable <breakpoint_id>
: Activates a specific breakpoint.<strong>disable <breakpoint_id></strong>
: Deactivates a specific breakpoint.debug <expression>
: Evaluates and prints the value of an expression.
These commands, along with standard pdb commands, enhance debugging with PDP++, facilitating code navigation, variable inspection, and breakpoint management.
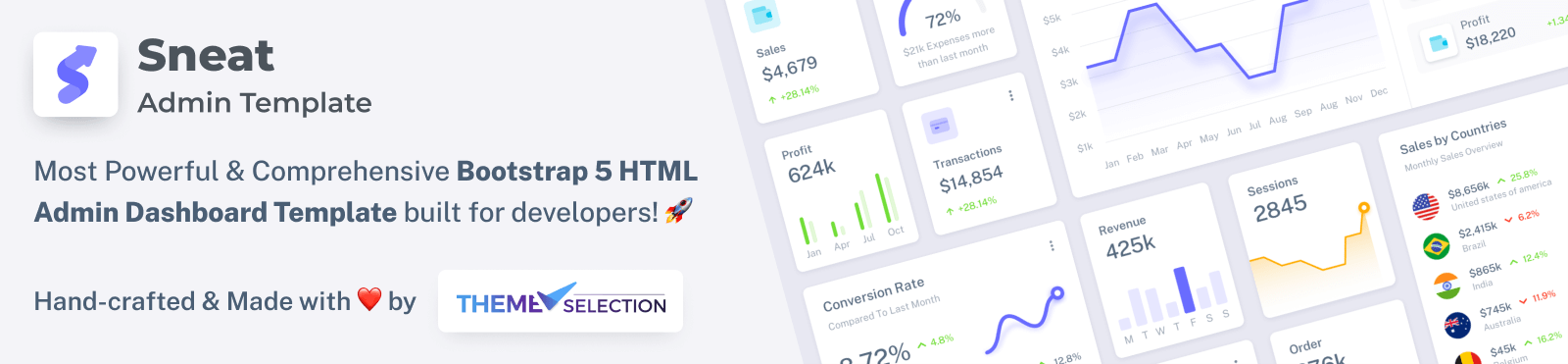
Ipdb
IPython pdb also known as iPDB is a valuable debugging tool for Python developers. It integrates the Python debugger (pdb) with the IPython shell, allowing for a seamless debugging experience. With IPython pdb, developers can launch the debugger from the IPython prompt and easily switch between debugging and exploring their code interactively.
It offers essential debugging capabilities like setting breakpoints, stepping through code, and examining variables, enabling developers to quickly identify and resolve issues in their Python programs. IPython pdb enhances the debugging workflow, providing a more efficient and productive debugging experience for Python developers. Thus, this can be considered the best Python Debuggers.
How To Use Ipdb?
ipdb exports function to access the IPython debugger, which features tab completion, syntax highlighting, better tracebacks, and better introspection with the same interface as the pdb module.
To use IPDB, you can follow these steps:
- Install IPDB:
<strong>pip install ipdb</strong>
- In your Python code, import IPDB by adding the
<strong>import ipdb</strong>
at the top. - Set breakpoints: To pause the execution of your code at a specific point, you can set breakpoints. Place the
<strong>ipdb.set_trace()</strong>
at the desired location in your code. - To start an interactive shell from the shell itself execute:
<strong>$ python -m ipdb Run-Script.py</strong>
- Debug your code: When your code reaches the line with the
<strong>ipdb.set_trace()</strong>
statement, it will pause the execution and drop you into the IPDB debugger shell. From there, you can use various commands to inspect variables, step through your code, and analyze the program’s state. - Continue or exit debugging: Once you have inspected and debugged your code, you can continue the execution by typing
c
orcontinue
in the IPDB shell. If you want to exit the debugger without continuing, typeq
orquit
.
Essential Commands:
Some of the essential and common ipdb commands that you are going to use in ipdb> shell. Most of the commands are the same as in pdb
and can this section can be skipped if you are acquainted with them.
- n[ext] :
n
simply continues program execution to the next line in the current method - s[tep] :
s
steps to the very next line of executable code, whether it is inside a called method or just on the next line
On a line where a method is being called, n
will “step over” the method execution code while s
will “step into” the method execution code allowing you to introspect the method code.
- w[here] :
w
prints a stack trace, with the most recent frame at the bottom. An arrow indicates the “current frame”, which determines the context of most commands. - b[reak]([filename:]lineno|function) :
b
adds breakpoints to the specified locations. Example usage:b sample-filename.py:<line no>
b <function>
b <lineno>
(for the current file)
- c[ontinue] :
c
continues program execution until another breakpoint is hit or the program execution completes - a[rgs] :
a
prints out all the arguments the current function received - r[eturn] :
r
continues execution until the current function returns
In case you are already using variables with names such as c
, a
use complete command continue
, args
to get the desired operation.
For more advanced usage of these commands refer to pdb’s documentation
Madbg
Madbg is a fully-featured remote debugger for Python. It provides a full remote tty, allowing sending keyboard signals to the debugger, tab completion, command history, line editing, and more.
It also runs the IPython debugger with all its capabilities. Additionally, madbg allows attaching to running programs preemptively (does not require gdb, unlike similar tools).
Madbg can be helpful for Python developers in debugging in a number of ways.
- Provides a full remote tty, allowing sending keyboard signals to the debugger, tab completion, command history, line editing, and more
- Runs the IPython debugger with all its capabilities
- Allows attaching to running programs preemptively (does not require gdb, unlike similar tools)
- Affects the debugged program minimally, although not yet recommended for use in production environments
- Provides TTY features even when the debugged program is a deamon, or runs outside a terminal
Here are some specific examples of how madbg can be used to debug Python code:
- You can use madbg to step through your code line by line, which can help you to identify the line of code that is causing the problem.
- You can use madbg to inspect variables, which can help you to understand the values of variables at different points in your code.
- You can use madbg to set breakpoints, which can help you to stop the execution of your code at a specific point so that you can inspect the state of your program.
- You can use madbg to attach to running programs preemptively, which can help you to debug problems that occur in production.
Thus, you can consider it as one of the best Python Debuggers.
Requires:
- Python >=3.7
How To Use madbg?
- To install madbg, use the
pip install madbg
command - To run a Python file with automatic post-mortem:
<strong>madbg run path_to_your_script.py</strong>
- To run a Python module similar to Python -m:
madbg run -m module.name
- To start a script, start the debugger from the first line:
madbg run --use-set-trace script.py
Refer to the installation process on official documentation.
Conclusion
In conclusion, numerous exceptional Python debuggers exist, each catering to different needs and preferences. For those seeking a straightforward and user-friendly debugger, the Python standard debugger (PDB) proves to be a commendable choice. However, individuals requiring a more robust and feature-laden debugger can explore a plethora of third-party options, including PyCharm, Visual Studio Code, and Sentry.
Regardless of the selected debugger, it is imperative to invest time in acquiring effective proficiency. Debugging is a time-intensive undertaking, yet its significance in ensuring the quality of Python code cannot be overstated. Utilizing a debugger enables swift identification and resolution of errors, thereby saving valuable time and mitigating frustration in the long term.
Here are some additional tips for debugging Python code:
- Use a debugger to step through your code line by line. This will allow you to see exactly what is happening at each step.
- Set breakpoints at key points in your code. This will allow you to stop execution at specific points and inspect the state of your program.
- Use the debugger’s variable inspector to view the values of variables. This can be helpful for tracking down errors caused by incorrect variable values.
- Use the debugger’s call stack to see the current execution context. This can be helpful for tracking down errors caused by function calls.
By following these tips, you can make the debugging process easier and more efficient.